longest_substring_without_repeating_characters
这题用到了哈希表 和 滑动窗口
滑动窗口:
就是有两个变量分别指示 窗口的 头和尾,就可以任意改变这两个变量
哈希表:
哈希表存着目前最长子组的值-地址对
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
| #include<iostream> #include<unordered_map> #include<vector> using namespace std;
class Solution { private: public: int lengthOfLongestSubstring(string s); }; int Solution::lengthOfLongestSubstring(string s) { int32_t begin=0,end=0,begin_new=0; unordered_map<char,int> windows; int count=0; for (size_t i = 0; i < s.size(); i++) { if(windows.count(s[end])==0) { windows.insert(make_pair(s[end],end)); end++; } else { if (end-begin>count || count==0) { count=end-begin; } begin_new=windows[s[end]]+1; for (size_t i = begin; i < begin_new; i++) { windows.erase(s[i]); } windows.insert(make_pair(s[end],end)); end++; begin=begin_new; } } if (end-begin>count) { count=end-begin; } return count; }
|
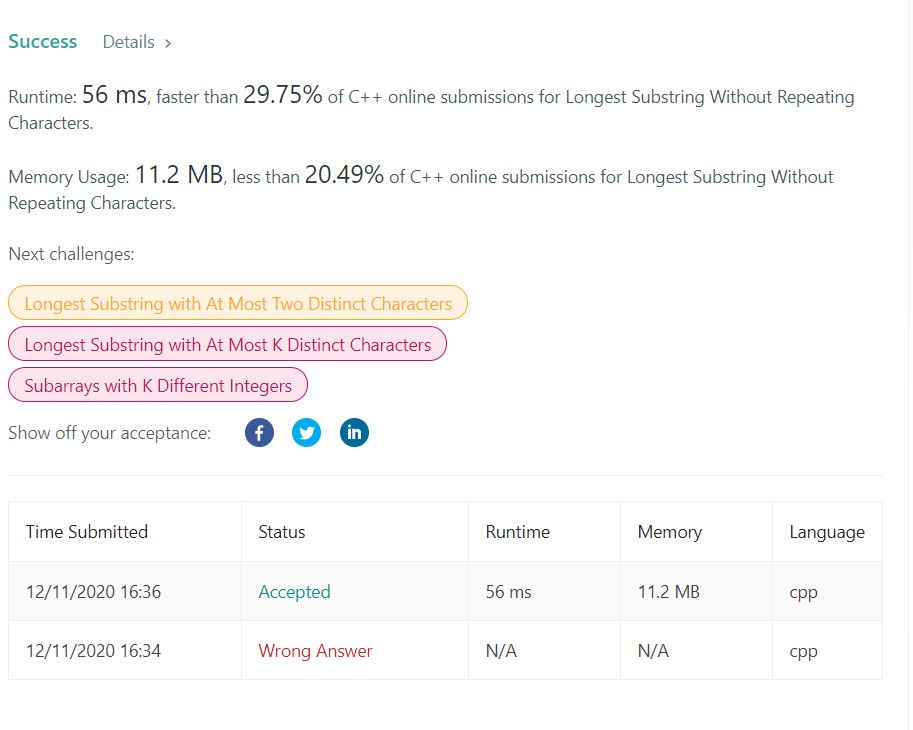